Create an email address from a name
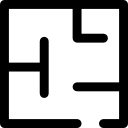
This a problem set for you to work through [1]
This is a problem set. Some of these are easy, others are far more difficult. The purpose of these problems sets are:
- to build your skill applying computational thinking to a problem
- to assess your knowledge and skills of different programming practices
What is this problem set trying to do[edit]
In this example we are primarily learning about strings and processing user input.
The Problem[edit]
This problem set is used with enormous gratitude from CS50[2]
Design and implement a program, that, given a person’s name, prints an email address.
- Your program will ask for a full first and full last name
- Your program will then create an email address with the following rules:
- the first letter of the email shall be the lower case first letter of the users first name. For example, Bill MacKenty would result in a 'b' here.
- the next string of characters shall be the full last name, all lowercase with no spaces For example, Bill MacKenty, would result in 'mackenty'.
- the suffix would then be added to the string: '@aswarsaw.org'.
Unit Tests[edit]
- User Input: Name: Bill MacKenty
- Expected output: bmackenty@aswarsaw.org
- User Input: Name: Jan Nowak
- Expected output: jnowak@aswarsaw.org
- User Input: Name: Buzz Lightyear
- Expected output: blighyear@aswarsaw.org
Hacker edition[edit]
In the hacker version:
- Ask the user for their year of graduation and add that to the start of the email.
- for example, if a student graduates in 2019, and their full name is Bill MacKenty, the output would be: 19bmackenty@aswarsaw.org
How you will be assessed[edit]
Your solution will be graded using the following axis:
Scope
- To what extent does your code implement the features required by our specification?
- To what extent is there evidence of effort?
Correctness
- To what extent did your code meet specifications?
- To what extent did your code meet unit tests?
- To what extent is your code free of bugs?
Design
- To what extent is your code written well (i.e. clearly, efficiently, elegantly, and/or logically)?
- To what extent is your code eliminating repetition?
- To what extent is your code using functions appropriately?
Style
- To what extent is your code readable?
- To what extent is your code commented?
- To what extent are your variables well named?
- To what extent do you adhere to style guide?
References[edit]
A possible solution[edit]
Click the expand link to see one possible solution, but NOT before you have tried and failed!
#This program inputs first name, last name , graduation year(if applicable) then it ouputs the ASW email
#It will use the same system as ASW (i.e. Hung Cuong Nguyen Duc graduating 2020 will be 20nguyenduc_h@aswarsaw.org)
#Created by Filip a grade 11 student
#Last modified 10.4.19
def teacher_email(first_name,last_name):
last_name = last_name.replace(" ","")
email = first_name.lower()[0] + last_name.lower() + "@aswarsaw.org"
return email
def student_email(grad_year, first_name, last_name):
last_name = last_name.replace(" ","")
email = grad_year[-2:] + last_name.lower() + "_" + first_name.lower()[0] + "@aswarsaw.org"
return email
position = input("Enter [T] if you are a teacher and enter [S] if you are a student: ")
if position != "S":
if position != "T":
print("Error: please enter [T] or [S] only.")
exit()
first_name = input("Enter your first name: ")
last_name = input("Enter your last name: ")
if position == "T":
print("Your ASW email is " + teacher_email(first_name, last_name))
elif position == "S":
grad_year = input("Enter your graduation year: ")
print("Your ASW email is " + student_email(grad_year,first_name,last_name))