Guess a number
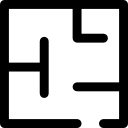
This is a problem set. Some of these are easy, others are far more difficult. The purpose of these problems sets are to HELP YOU THINK THROUGH problems. The solution is at the bottom of this page, but please don't look at it until you have tried (and failed) at least three or four times.
What is this problem set trying to do[edit]
You have to think about conditionals and computational thinking here. You are also going to be incrementing variables.
The Problem[edit]
Please write a simple game that randomly selects a number between 0 and 100. A player must guess the number. Your program should tell the player if their guess is higher or lower than the secret number.
- Your program must generate a random number between 0 and 100 and assign that random number to a variable.
- Your program must ask the player to guess a number
- Your program must compare the players guess and the secret number. The program should tell the player if their guess is higher or lower than the secret number
- Your program must tell the player if they have won
- Your program must ask the player if they want to play again
Some Python code to get you started[edit]
This code will not run. If you see ...
I have left out code, and you have to figure out what to put in.
import random
secret_number = ...
guess = 0
game = 1
print("This is guess: " + str(guess))
print("This is game:" + str(game))
player_guess = input("Please make a guess: ")
guess = guess +1
...
play_again = input("Do you want to play again? ")
if play_again == "y" or play_again == "Y" or play_again == "yes":
guess = 0
game = game + 1
break
....
Take This Further[edit]
- add in a condition that players only have a certain number of turns to win
- add in a difficulty level; easy, medium and expert. If a player has easy, they have 8 tries, medium, they have only 4 tries, and expert, only 2 tries!!
- you can tell a player if their guess is "a little to high or a little to low" or you can say "your guess is way to high or way to low"
How you will be assessed[edit]
Every problem set is a formative assignment. Please click here to see how you will be graded
References[edit]
A possible solution[edit]
Click the expand link to see one possible solution, but NOT before you have tried and failed!
#Finished on 4/10/18
#Can catch errors and let users input again
#Author: Filip
import random
secret_number = random.randint(1, 100)
counter = 0
while True:
try:
difficulty_level = int(input("What level difficultly level do you want(1-easiest 5-impossible): "))
if difficulty_level < 1 or difficulty_level > 5:
raise NameError()
except ValueError:
print("ERROR: You can only input numbers!")
continue
except NameError:
print("ERROR: Please input a number between 1 and 5.")
continue
else:
if difficulty_level == 1:
turns_left = 15
elif difficulty_level == 2:
turns_left = 12
elif difficulty_level == 3:
turns_left = 10
elif difficulty_level == 4:
turns_left = 7
elif difficulty_level == 5:
turns_left = 5
while counter<turns_left:
try:
user_guess = int(input("What do you think the number is (1-100): "))
except ValueError:
print("ERROR: You can only input numbers!")
else:
if user_guess<101 and user_guess > 0:
if user_guess>secret_number:
counter += 1
if user_guess-secret_number <4:
print("GUESS A LITTLE LOWER")
else:
print("GUESS LOWER")
elif user_guess<secret_number:
counter += 1
if secret_number-user_guess <4:
print("GUESS A LITTLE HIGHER")
else:
print("GUESS HIGHER")
else:
counter += 1
print("Good job!! It took you " + str(counter) + " tries.")
break
else:
print("ERROR: Your number has to be between 1 and 100!")
if counter>=turns_left:
print("GAME OVER: You ran out of turns")
break