Connecting to underlying data sources: Difference between revisions
Mr. MacKenty (talk | contribs) No edit summary |
Mr. MacKenty (talk | contribs) No edit summary |
||
(2 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
[[file:Connection.png|right|frame|Web Science<ref>http://www.flaticon.com/</ref>]] | [[file:Connection.png|right|frame|Web Science<ref>http://www.flaticon.com/</ref>]] | ||
== Connecting Web Pages to Underlying Data Sources == | |||
Web pages can connect to underlying data sources, such as databases, using server-side scripting languages like PHP. This enables web pages to interact with databases to retrieve or store data and dynamically generate content based on user inputs or application requirements. | |||
=== Steps to Connect a Web Page to a Database === | |||
<syntaxhighlight lang="PHP"> | # Setting up the Database: | ||
A database (e.g., MySQL, MariaDB, PostgreSQL) is used to store structured data. For example, a database for a product catalog might have a table named `products` with columns like `id`, `name`, `description`, and `price`. | |||
# Creating the Connection: | |||
PHP establishes a connection to the database using extensions such as `mysqli` or `PDO`. This connection requires credentials like the server address, username, password, and database name. | |||
# Querying the Database: | |||
SQL (Structured Query Language) is used to perform operations like fetching, inserting, updating, or deleting data. PHP sends SQL queries to the database. | |||
# Processing and Displaying Data: | |||
The results of SQL queries are processed in PHP and displayed on the web page using HTML. | |||
=== Example Code: Connecting a Web Page to a MySQL Database === | |||
==== Step 1: Database Setup ==== | |||
Create a MySQL database and table: | |||
<syntaxhighlight lang="sql"> | |||
CREATE DATABASE web_app; | |||
USE web_app; | |||
CREATE TABLE products ( | |||
id INT AUTO_INCREMENT PRIMARY KEY, | |||
name VARCHAR(255) NOT NULL, | |||
description TEXT, | |||
price DECIMAL(10, 2) | |||
); | |||
INSERT INTO products (name, description, price) VALUES | |||
('Laptop', 'A powerful laptop', 1200.99), | |||
('Smartphone', 'A sleek new smartphone', 699.50), | |||
('Headphones', 'Noise-cancelling headphones', 199.99); | |||
</syntaxhighlight> | |||
==== Step 2: PHP Code to Connect and Display Data ==== | |||
<syntaxhighlight lang="php"> | |||
<?php | <?php | ||
$ | // Include database configuration | ||
// | $server = "localhost"; | ||
if ( | $username = "root"; | ||
{ | $password = ""; | ||
$database = "web_app"; | |||
// Create a database connection | |||
$conn = new mysqli($server, $username, $password, $database); | |||
// Check the connection | |||
if ($conn->connect_error) { | |||
die("Connection failed: " . $conn->connect_error); | |||
} | } | ||
// Fetch data from the 'products' table | |||
$sql = "SELECT name, description, price FROM products"; | |||
$result = $conn->query($sql); | |||
< | // Display the data | ||
if ($result->num_rows > 0) { | |||
echo "<h1>Product Catalog</h1><ul>"; | |||
while ($row = $result->fetch_assoc()) { | |||
echo "<li><strong>" . $row["name"] . "</strong>: " . $row["description"] . " ($" . $row["price"] . ")</li>"; | |||
} | |||
echo "</ul>"; | |||
} else { | |||
echo "No products found."; | |||
} | |||
// Close the connection | |||
$ | $conn->close(); | ||
?> | ?> | ||
</syntaxhighlight> | </syntaxhighlight> | ||
This example demonstrates a simple PHP script connecting to a MySQL database, fetching data from a `products` table, and displaying it in an HTML format. | |||
=== Summary === | |||
Using PHP, you can seamlessly connect web pages to databases, enabling dynamic and interactive web applications. This process involves setting up a database, connecting to it using PHP, querying the data, and displaying it on the web page. | |||
== Standards == | == Standards == |
Latest revision as of 08:38, 3 December 2024
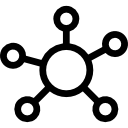
Connecting Web Pages to Underlying Data Sources[edit]
Web pages can connect to underlying data sources, such as databases, using server-side scripting languages like PHP. This enables web pages to interact with databases to retrieve or store data and dynamically generate content based on user inputs or application requirements.
Steps to Connect a Web Page to a Database[edit]
- Setting up the Database:
A database (e.g., MySQL, MariaDB, PostgreSQL) is used to store structured data. For example, a database for a product catalog might have a table named `products` with columns like `id`, `name`, `description`, and `price`.
- Creating the Connection:
PHP establishes a connection to the database using extensions such as `mysqli` or `PDO`. This connection requires credentials like the server address, username, password, and database name.
- Querying the Database:
SQL (Structured Query Language) is used to perform operations like fetching, inserting, updating, or deleting data. PHP sends SQL queries to the database.
- Processing and Displaying Data:
The results of SQL queries are processed in PHP and displayed on the web page using HTML.
Example Code: Connecting a Web Page to a MySQL Database[edit]
Step 1: Database Setup[edit]
Create a MySQL database and table:
CREATE DATABASE web_app;
USE web_app;
CREATE TABLE products (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
description TEXT,
price DECIMAL(10, 2)
);
INSERT INTO products (name, description, price) VALUES
('Laptop', 'A powerful laptop', 1200.99),
('Smartphone', 'A sleek new smartphone', 699.50),
('Headphones', 'Noise-cancelling headphones', 199.99);
Step 2: PHP Code to Connect and Display Data[edit]
<?php
// Include database configuration
$server = "localhost";
$username = "root";
$password = "";
$database = "web_app";
// Create a database connection
$conn = new mysqli($server, $username, $password, $database);
// Check the connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Fetch data from the 'products' table
$sql = "SELECT name, description, price FROM products";
$result = $conn->query($sql);
// Display the data
if ($result->num_rows > 0) {
echo "<h1>Product Catalog</h1><ul>";
while ($row = $result->fetch_assoc()) {
echo "<li><strong>" . $row["name"] . "</strong>: " . $row["description"] . " ($" . $row["price"] . ")</li>";
}
echo "</ul>";
} else {
echo "No products found.";
}
// Close the connection
$conn->close();
?>
This example demonstrates a simple PHP script connecting to a MySQL database, fetching data from a `products` table, and displaying it in an HTML format.
Summary[edit]
Using PHP, you can seamlessly connect web pages to databases, enabling dynamic and interactive web applications. This process involves setting up a database, connecting to it using PHP, querying the data, and displaying it on the web page.
Standards[edit]
These standards are used from the IB Computer Science Subject Guide[2]
- Describe how web pages can be connected to underlying data sources.
References[edit]
- ↑ http://www.flaticon.com/
- ↑ IB Diploma Programme Computer science guide (first examinations 2014). Cardiff, Wales, United Kingdom: International Baccalaureate Organization. January 2012.