Modelling a simple system
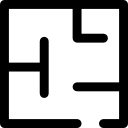
This is a problem set. Some of these are easy, others are far more difficult. The purpose of these problems sets are:
- to build your skill applying computational thinking to a problem
- to assess your knowledge and skills of different programming practices
What is this problem set trying to do[edit]
This problem set is linked to modeling and Simulation. We are applying our knowledge and understanding of dictionaries, conditionals, computational thinking & problem-solving and iteration.
Please understanding how this simulation works.
The Problem[edit]
This problem set has four different parts.We are starting simple and working our way up to more complexity.
Part 1[edit]
- An ant is a little insect that generally isn't very cute and likes to eat things.
- An ant has an ID number. Each ID number is unique
- An ant has a boolean state named life: alive or dead
- An ant has a boolean state named mode : looking for food, bringing food to nest
Create this model in python using a dictionary data type. Please save your file as ants.py. Please have two ants. Warning! Because this dictionary is going hold many ants, we need to use a nested dictionary. Please print the full dictionary for each ant.
Part 2[edit]
- An ant should ALSO have a boolean state "moving" : yes or no
- An ant should ALSO have an attribute "movement direction": North, South, West, East
Add these two states to each of your ants. Like before, please print the full dictionary for each ant.
Part 3[edit]
- An ant should ALSO have a state which describes it's current position in X,Y coordinates:
- current position x : an integer between 0 and 100 for X position
- current position y : an integer between 0 and 100 for Y position
Add these these two states to each of your ants. Like before, please print the full dictionary for each ant.
Part 4[edit]
- Create a fixed-count loop. The loop should range from 0 to 10.
- Each iteration should represent one second.
- Each iteration, print the following attributes for each ant:
- moving?
- moving direction?
- alive?
- mode?
- current position X?
- current position Y?
Please don't complicate this. I just want to see textual output of 10 seconds, please. Also, don't worry about updating the position of the ant. I just need you to see what 10 seconds for our model looks like.
How you will be assessed[edit]
Your solution will be graded using the following axis:
Scope
- To what extent does your code implement the features required by our specification?
- To what extent is there evidence of effort?
Correctness
- To what extent did your code meet specifications?
- To what extent did your code meet unit tests?
- To what extent is your code free of bugs?
Design
- To what extent is your code written well (i.e. clearly, efficiently, elegantly, and/or logically)?
- To what extent is your code eliminating repetition?
- To what extent is your code using functions appropriately?
Style
- To what extent is your code readable?
- To what extent is your code commented?
- To what extent are your variables well named?
- To what extent do you adhere to style guide?
References[edit]
A possible solution[edit]
Click the expand link to see one possible solution, but NOT before you have tried and failed!
# ========= PART ONE ============
ants = {
1: {
"life": "alive",
"state": "looking for food"
},
2: {
"life": "alive",
"state": "bringing food to nest"
}
}
print("Ant 1 is: ", ants[1])
print("Ant 2 is: ", ants[2])
# ================ PART TWO ===============
ants = {
1: {
"life": "alive",
"state": "looking for food",
"moving": "yes",
"movement direction": "North"
},
2: {
"life": "alive",
"state": "bringing food to nest",
"moving": "yes",
"movement direction": "North"
}
}
print("Ant 1 is: ", ants[1])
print("Ant 2 is: ", ants[2])
# ======== PART THREE =========
ants = {
1: {
"life": "alive",
"state": "looking for food",
"moving": "yes",
"movement direction": "North",
"current position x": 0,
"current position y": 0
},
2: {
"life": "alive",
"state": "bringing food to nest",
"moving": "yes",
"movement direction": "North",
"current position x": 100,
"current position y": 100
}
}
print("Ant 1 is: ", ants[1])
print("Ant 2 is: ", ants[2])
# ======== PART FOUR =========
ants = {
1: {
"life": "alive",
"state": "looking for food",
"moving": "yes",
"movement direction": "North",
"current position x": 0,
"current position y": 0
},
2: {
"life": "alive",
"state": "bringing food to nest",
"moving": "no",
"movement direction": "North",
"current position x": 100,
"current position y": 100
}
}
print("Ant 1 is: ", ants[1])
print("Ant 2 is: ", ants[2])
for i in range(0,5):
print("=== Second ", i, "===")
for j in ants.keys(): # we need to get the size of the dictionary
print("Ant ", j, " moving: ", ants[j]["moving"])
print("Ant ", j, " moving direction: ", ants[j]["movement direction"])
print("Ant ", j, " life: ", ants[j]["life"])
print("Ant ", j, " state: ", ants[j]["state"])
print("Ant ", j, " current position x ", ants[j]["current position x"])
print("Ant ", j, " current position y: ", ants[j]["current position y"])