Programming: Difference between revisions
Mr. MacKenty (talk | contribs) (Created page with "<center> <blockquote style="padding: 5px; background-color: #FFF8DC; border: solid thin gray;"> File:Exclamation.png This is one of '''the most important ideas''' you c...") |
Mr. MacKenty (talk | contribs) |
||
(81 intermediate revisions by 2 users not shown) | |||
Line 1: | Line 1: | ||
[[file:computation.png|right|frame|Programming<ref>http://www.flaticon.com/</ref>]] | |||
= Introduction to programming = | |||
Programming is the process of planning, writing, executing and testing instructions for a computer system. | |||
== | * [[What is a programming language?]] [[File:Answer.png|This topic has formative assessment as part of the article.]]<ref name="formative">Icons made by https://www.flaticon.com/authors/eucalyp from https://www.flaticon.com/ </ref> | ||
** [[Fundamental and compound operations]] | |||
** [[Higher level and lower level languages]] [[File:Answer.png|This topic has formative assessment as part of the article.]]<ref name="formative">Icons made by https://www.flaticon.com/authors/eucalyp from https://www.flaticon.com/ </ref> | |||
** [[Interpreted and compiled languages]] | |||
=== | == What is the best programming language? == | ||
<html> | |||
<iframe width="560" height="315" src="https://www.youtube.com/embed/RfWGJS7rckk" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe> | |||
</html> | |||
* [[ | == Learning to Program == | ||
* [[ | * [[Inputs and outputs]] | ||
* [[ | * [[Variables]] [[File:Answer.png|This topic has formative assessment as part of the article.]]<ref name="formative">Icons made by https://www.flaticon.com/authors/eucalyp from https://www.flaticon.com/ </ref> | ||
* [[Functions]] | |||
* [[Data types]] [[File:Answer.png|This topic has formative assessment as part of the article.]]<ref name="formative">Icons made by https://www.flaticon.com/authors/eucalyp from https://www.flaticon.com/ </ref> | |||
* [[Iteration]] | |||
* [[Conditionals | Selection]] | |||
* [[Operators]] | |||
== Primitive data types == | |||
In computer science and computer programming, a data type or simply type is a classification of data which tells the compiler or interpreter how the programmer intends to use the data. Most programming languages support various types of data, for example: real, integer or Boolean.<ref>https://en.wikipedia.org/wiki/Data_type</ref> | |||
The list below describes some of the more common primitive data types | |||
* [[ | * [[Scalar type]] | ||
* [[Boolean]] | |||
* [[Int|Integers]] | |||
** [[Signed integers]] | |||
** [[Unsigned integers]] | |||
* [[Float]] | |||
* [[Char]] | |||
* [[String]] | |||
== | == Common data structures == | ||
A data structure is just some organization of data that we've built into an orderly arrangement. The organization and arrangement of data can make our programs run much more efficiently. Each data structure has advantages and disadvantages. There are common data structures and abstract data structures. In general abstract data structures are advanced and more specific to a specific task. | |||
=== | === Common data structures which are assessed by the IB === | ||
* [[ | * [[Arrays]] | ||
* [[ | * [[two-dimensional arrays]] | ||
* [[ | * [[Collections]] | ||
* [[ | * [[Linked list]] | ||
* [[Objects]] | |||
=== | === Common data structures which are not assessed by the IB === | ||
* [[ | * [[Lists]] | ||
* [[ | * [[Dictionaries]] | ||
* [[ | * [[Sets]] | ||
* [[ | * [[Tuple]] | ||
=== | === Abstract data structures which are assessed by the IB === | ||
For a deeper understanding of abstract data structures, please see this page: [[Abstract data structures]] | |||
* [[stack]] | |||
* [[queue]] | |||
* [[linked list]] | |||
* [[tree]] | |||
* [[binary tree]] | |||
* [[ | = Python = | ||
* Please visit our [[python]] programming page | |||
* [[ | = Programming Paradigms = | ||
* [[ | * [[Object-Oriented Programming]] ([[Separation of concerns]]) | ||
* [[ | * [[Procedural programming]] | ||
* [[ | * [[Declarative programming]] | ||
* [[ | * [[Functional programming]] | ||
* [[Imperative programming]] | |||
= | = Advanced Programming = | ||
* [[Regular expressions]] | * [[Regular expressions]] | ||
* [[ | * [[Artificial Intelligence]] | ||
* [[ | * [[API]] | ||
* [[Robotics]] | |||
* [[Working with files]] | |||
* Working with [[Databases|databases]] | |||
* [[GUI|Graphical User Interfaces]] | |||
* [[Hashing]] | |||
* [[Blockchain]] | |||
* [[Genetic Algorithms]] | |||
* [[Machine learning]] | |||
== References == | == References == | ||
<references /> | <references /> | ||
[[Category:programming]] | [[Category:programming]] |
Latest revision as of 09:11, 10 October 2023
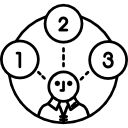
Introduction to programming[edit]
Programming is the process of planning, writing, executing and testing instructions for a computer system.
What is the best programming language?[edit]
Learning to Program[edit]
Primitive data types[edit]
In computer science and computer programming, a data type or simply type is a classification of data which tells the compiler or interpreter how the programmer intends to use the data. Most programming languages support various types of data, for example: real, integer or Boolean.[3]
The list below describes some of the more common primitive data types
Common data structures[edit]
A data structure is just some organization of data that we've built into an orderly arrangement. The organization and arrangement of data can make our programs run much more efficiently. Each data structure has advantages and disadvantages. There are common data structures and abstract data structures. In general abstract data structures are advanced and more specific to a specific task.
Common data structures which are assessed by the IB[edit]
Common data structures which are not assessed by the IB[edit]
Abstract data structures which are assessed by the IB[edit]
For a deeper understanding of abstract data structures, please see this page: Abstract data structures
Python[edit]
- Please visit our python programming page
Programming Paradigms[edit]
- Object-Oriented Programming (Separation of concerns)
- Procedural programming
- Declarative programming
- Functional programming
- Imperative programming
Advanced Programming[edit]
- Regular expressions
- Artificial Intelligence
- API
- Robotics
- Working with files
- Working with databases
- Graphical User Interfaces
- Hashing
- Blockchain
- Genetic Algorithms
- Machine learning